How To Use Switch Statement In Dev C++
You can use the switch statement in C++ to make choices between options. The following SwitchCalculator program uses the switch statement to implement a simple calculator:
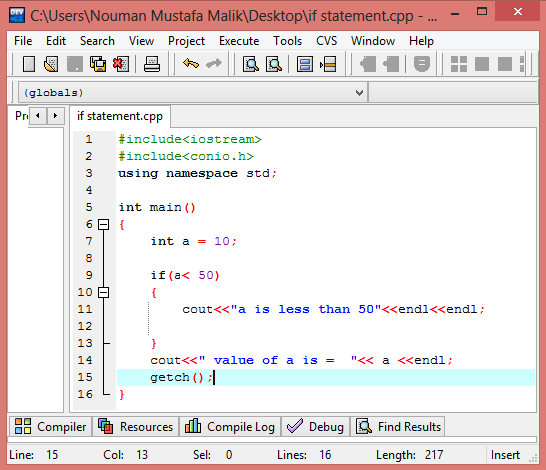
This program begins by prompting the user to enter “value1 op value2” where op is one of the common arithmetic operators +, -, *, / or %. The program then reads the variables nOperand1, cOperator, and nOperand2.
A switch statement can be nested. In such cases, case or default labels associate with the closest switch statement that encloses them. Microsoft Specific. Microsoft C does not limit the number of case values in a switch statement. The number is limited only by the available memory. ANSI C requires at least 257 case labels be allowed in a.
The program starts by echoing back to the user what it read from the keyboard. It follows this with the result of the calculation.

Echoing the input back to the user is always a good programming practice. It gives the user confirmation that the program read his input correctly.
The switch on cOperator differentiates between the operations that this calculator implements. For example, in the case that cOperator is ‘+’, the program reports the sum of nOperand1 and nOperand2.
Jun 03, 2015 switch case is a branching statement used to perform action based on available choices, instead of making decisions based on conditions. Using switch case you can write more clean and optimal code than if else statement. Switch case only works with integer, character and enumeration constants. In this exercises we will focus on the use of switch case statement. Mar 19, 2020 Welcome to the 5th tutorial of this tutorial series for beginners in C. We are going to have some more fun with the control structures in this part. We will introduce you to the “Switch” statement and in the later part will move to the iteration structures such as “for”, “while”, “do while” loops. After doing that it uses the switch case statement, to check if the variable called name2 is 1 or 2 or 3 or 4. After that its adds 1 into counter and shows me the result. The problem currently is that it is not showing anything other then all values = 0. Dec 22, 2008 Using Range in the Case Values of Switch Statement C Programming Video Tutorial - Duration: 12:07. LearningLad 16,202 views. Shapes in c using nested loops and pattern. C: Switch Statements Sometimes when creating a C program, you run into a situation in which you want to compare one thing to a number of other things. Let's say, for example, that you took a character from the user and wanted to compare this to a number of characters to perform different actions. C switch statement - A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is chec.
Because ‘X’ is another common symbol for multiply, the program accepts ‘*’, ‘X’, and ‘x’ all as synonyms for multiply using the case “fall through” feature. The program outputs an error message if cOperator doesn’t match any of the known operators.
The output from a few sample runs appears as follows:
Notice that the final run executes the default case of the switch statement since the character ‘$’ did not match any of the cases.
- C Programming Tutorial
- C Programming useful Resources
- Selected Reading
A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each switch case.
Syntax
The syntax for a switch statement in C programming language is as follows −
How To Use Switch Statement In Dev C Pdf
The following rules apply to a switch statement −
The expression used in a switch statement must have an integral or enumerated type, or be of a class type in which the class has a single conversion function to an integral or enumerated type.
You can have any number of case statements within a switch. Each case is followed by the value to be compared to and a colon.
The constant-expression for a case must be the same data type as the variable in the switch, and it must be a constant or a literal. Cooking and serving games free download.
When the variable being switched on is equal to a case, the statements following that case will execute until a break statement is reached.
When a break statement is reached, the switch terminates, and the flow of control jumps to the next line following the switch statement.
Not every case needs to contain a break. If no break appears, the flow of control will fall through to subsequent cases until a break is reached.
The three-month plan is intended for the intermediate user, who has a certain skill with Traktor and wants to move forward towards mid - advanced use in a short time and can practice in a daily basis. Jun 19, 2013 Pro tip: Traktor’s crossfader curve can be adjust in the Preferences. Click the cogwheel again and click on Mixer. Click the cogwheel again and click on Mixer. There you can adjust the crossfader curve from smooth to sharp, as well as set the auto-crossfade time and the EQ type. How to use traktor pro 2 for beginners. Mar 23, 2016 PB DJ instructor Dave Clarke looks at mixing using Traktor Pro DJ. Sample our online Traktor course for free here: P. Mar 04, 2016 Point Blank DJ Instructor David Clarke runs through the basics of using Traktor in this tutorial which covers Importing Music, Analysing Tracks, Cue Points and more. Find out more about our online. Sep 08, 2014 Traktor Scratch Pro 2 is the DVS (digital vinyl system) version of Traktor Pro 2. If you want to use turntables or CDJs with Traktor, upgrade to this version. It’s identical to the flagship Traktor Pro 2, with the difference being this package includes two pieces of timecode vinyl and two pieces of timecode CDs for use with a pair of turntables or CDJs, respectively.
A switch statement can have an optional default case, which must appear at the end of the switch. The default case can be used for performing a task when none of the cases is true. No break is needed in the default case.
How To Use Switch In Dev C++
Flow Diagram
Example
When the above code is compiled and executed, it produces the following result −