Argc Argv Dev C++
The full declaration of main looks like this: The integer, argc is the ARGument Count (hence argc). It is the number of arguments passed into the program from the command line, including the name of the program.
I am beginner in C and I am used to code with int main, and now I am working with: int main(int argc, char.argv) And I don't know exactly what this line of code means. So, I looked up for some answer in the internet and I found this block of code. Learn how to accept command line arguments in C using the argv and argc parameters to main. Starting out How to begin. Command line arguments in C using argc and argv. Command-line arguments are given after the name of a program in command-line operating systems like DOS or Linux, and are passed in to the program from the operating. The name of the variable argv stands for 'argument vector'. A vector is a one-dimensional array, and argv is a one-dimensional array of strings. Each string is one of the arguments that was passed to the program. For example, the command line gcc -o myprog myprog.c would result in the following values internal to GCC: argc 4 argv0 gcc argv1. May 27, 2011 In this tutorial on C I want to show you the command line arguments. Actually we will give parameters to the main function. To use command line arguments in your program, you must first understand the full declaration of the main function, which previously has accepted no arguments. In fact, main can actually accept two arguments: one argument is number of command line arguments, and the other argument is a full list of all of the command line arguments.
The array of character pointers is the listing of all the arguments. argv[0] is the name of the program, or an empty string if the name is not available. After that, every element number less than argc is a command line argument. You can use each argv element just like a string, or use argv as a two dimensional array. argv[argc] is a null pointer.
How could this be used? Almost any program that wants its parameters to be set when it is executed would use this. One common use is to write a function that takes the name of a file and outputs the entire text of it onto the screen. This program is fairly simple. It incorporates the full version of main. Then it first checks to ensure the user added the second argument, theoretically a file name. The program then checks to see if the file is valid by trying to open it. This is a standard operation that is effective and easy. If the file is valid, it gets opened in the process. The code is self-explanatory, but is littered with comments, you should have no trouble understanding its operation this far into the tutorial. :-)
Quiz yourself
Previous: Functions Continued
Next: Linked Lists
Back to C++ Tutorial Index
In Unix, when you pass additional arguments to a command, those commands must be passedto the executing process. For example, in calling ls -al, it executes the programls and passes the string -al as an argument:
The way a running program accesses these additional parameters isthat these are passed as parameters to the function main:

Here argc means argument count andargument vector.
How To Use Argc And Argv
The first argument is the number of parameters passed plus one to include thename of the program that was executed to get those process running. Thus,argc is always greater than zero and argv[0] is the name ofthe executable (including the path) that was run to begin this process. For example, if we run
Here we compile this code and first compile and run it so that the executable name is a.out and thenwe compile it again and run it so the executable name is arg:
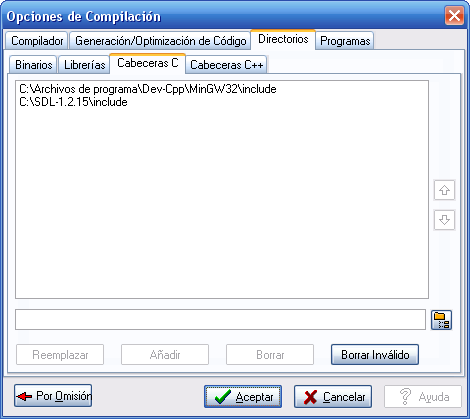
Argc Argv C++
If more additional command-line arguments are passed, the string of all characters is parsedand separated into substrings based on a few rules; however, if all the characters are eithercharacters, numbers or spaces, the shell will separate the based on spaces and assign args[1]the address of the first, args[2] the address of the second, and so on.
The following program prints all the arguments:
Here we execute this program with one and then twelve command-line arguments:
For Fun
Now, you may be wondering what actually happens (and if you don't, you're welcome to skip this).First, when the command is executed, the shellparses the command line and separates the individual pieces by a null character 0. For example,the execution of
New downloads are added to the member section daily and we now have 356,514 downloads for our members, including: TV, Movies, Software, Games, Music and More.It's best if you avoid using common keywords when searching for Refx Vanguard Vsti Rtas 1.8.0. Refx Vanguard Vsti Rtas 1.8.0 was added to DownloadKeeper this week and last updated on 15-Apr-2020. Nexus vst download.
From time to time, we also make lists with best plugins. An example is 8 best free drum kit VST plugins for finger drumming, offering our pick of the most useful drum machines. Or 10 free VST plugins to improve your music productions, with some of the best tools to get started into. Free VST Plugins for FL Studio. Instructions: Click each link below and look around each website for the download link or button. Do not install any suspicious software. Click the VST name or image to visit the website to download the free plugin. Combo Model F. VST is a software interface standard that allows you to load VST software synthesizer and effect 'plugins' in FL Studio. VST is in addition to the FL Studio 'native' plugin format. VST plugins generally come in two types, instruments (VSTi) that are designed to make sound and effects (VST) that are designed to process sound, although some can. Where can i download vst plugins for fl studio o 20.
has the shell generate the string ./a.out☐first☐second☐third☐fourth☐fifth☐where the box represents the null character. Next, memory for an array of six (argc) pointers is allocatedand these six pointers are assigned the first character of each of the strings into which the command line was parsed.Finally, memory is allocated for the stack and using 8-byte alignment, the two arguments are placed into the stack.This is shown in Figure 1.
Figure 1. The result of calling ./a.out first second third fourth fifth on the command line.
Argc Argv Dev C Download
Further Fun
Dev C++ 5.11
In Unix, the shell does further processing of the command. For example, if it finds wild cards that indicate filesin the current directory, it will attempt to expand those wild cards. These include ? for one unknowncharacter and * for any number of characters.
Argc Argv Dev C 2017
For example, the following examines the file in the current directory:
Similarly, you can tell the command line to treat spaces as part of one string or ignore wildcardsby either using the backslash before thespace or wildcard or surrounding the string by single or double quotes.
Finally, if you surround text with backticks, it will execute that first and then replace that withthe output of the command:
Guess what happens if you enter ./a.out `ls -al`.